Effective Practices for Quality Software Development
Written on
Chapter 1: Introduction to Programming Practices
In the realm of software development, programmers utilize various techniques to enhance their coding efficiency and produce high-quality software. For instance, some developers prioritize writing clear and maintainable code, while others focus on creating optimized and resource-efficient algorithms. These practices serve as valuable guidelines for all developers aiming to improve their software creation processes. Senior engineers often document these techniques to aid their teams.
Programming best practices represent a collection of accepted methods among developers; however, they are not strict regulations, meaning that some may choose to disregard them. Ignoring these well-known practices can lead to time-consuming challenges down the line. Consider the repercussions of neglecting a bug in your code—over time, unresolved issues can complicate the debugging process significantly.
Maintaining a checklist of best practices can greatly enhance the quality of your software and enrich your programming career. With over ten years of experience in various domains, including desktop, mobile, and web development, I've compiled a list of essential programming practices derived from my journey. These guidelines are applicable to any software project you undertake.
Section 1.1: Prioritize Bug Prevention
Software bugs are an unavoidable aspect of coding, yet early detection and resolution can mitigate their impact. Identifying issues during the developer testing phase or while coding can significantly reduce the likelihood of bugs. For example, as you implement algorithms, scrutinizing all possible edge cases can help prevent many errors.
However, in the fast-paced environment of modern software development, detecting bugs early can be challenging. Often, teams focus on fixing bugs after the initial development phase. The Flutter team exemplifies this by categorizing critical bugs.
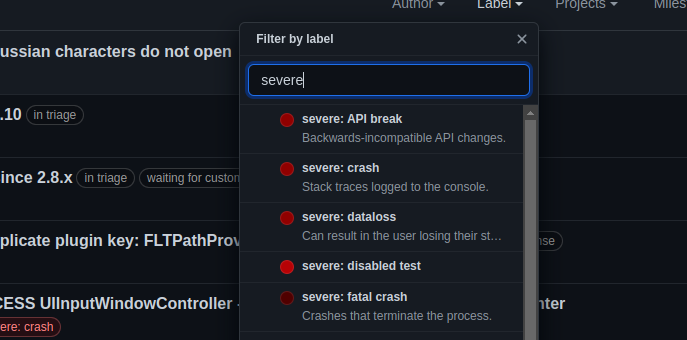
Code reviews typically emphasize style, design patterns, and syntax, which can allow bugs to remain hidden in the code. These concealed issues can escalate, requiring more time to rectify later. Always strive to prevent and resolve bugs to save valuable time in the future.
Section 1.2: Understand Before You Use Code Snippets
Official documentation often lacks specific code samples necessary for completing programming tasks. As a result, developers frequently turn to online forums, tutorials, and open-source projects for code snippets. However, these snippets may harbor bugs or compatibility issues.
It's crucial to thoroughly evaluate any code snippet before integrating it into your projects. Look for deprecated APIs, potential incompatibilities, and performance concerns. Additionally, ensure you comprehend the functionality of any code changes you make to avoid introducing new bugs or vulnerabilities.
Chapter 2: The Pursuit of Quality Code
Section 2.1: Aim for Good Code, Not Perfection
While the concept of "perfect code" is a myth, producing good code is very much achievable. Striving for perfection often results in over-engineered solutions. Instead, focus on writing code that is clean, efficient, and meets the necessary requirements.
Establish a clear code style guide and utilize appropriate data structures and design patterns. Refactor your code as needed to enhance maintainability, but avoid complicating it unnecessarily in the pursuit of perfection.
The following article provides insights on writing quality code using clean coding practices:
The first video title is "7 Coding Habits of Top 1% Programmers (that I wish I knew earlier)" — This video outlines key habits that can help improve coding skills significantly.
Section 2.2: Write Eco-Conscious Code
Can writing code contribute to environmental sustainability? Yes, it can. The generation of electricity, particularly from fossil fuels, often leads to environmental degradation. Moreover, more intensive computing generates excess heat and consumes more power. Research indicates that languages like C, Rust, and C++ are among the most resource-efficient.
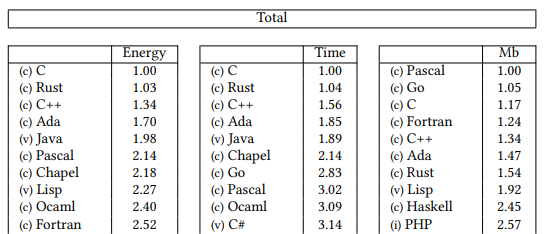
Writing optimized code that consumes less memory and CPU cycles ensures that your applications run efficiently without unnecessarily draining device resources. Always assess the time and space complexities of your algorithms, and select technologies that align with your efficiency goals.
Section 2.3: Build a Stable Core Before Adding Features
In a past experience, I embarked on an open-source project that required significant rewriting to ensure stability before new features could be added. Establishing a solid foundation is essential; an unstable core can lead to extensive rewrites later on. Concepts like the Minimum Viable Product (MVP) support this philosophy.
Many development teams separate core functionalities from feature modules to improve stability. For instance, Apple maintains the Darwin core for its operating systems, while features are added to iOS and macOS independently.
Section 2.4: Choose the Right Architecture and Technology
Software architecture plays a crucial role in organizing and structuring a software system. Selecting the appropriate architecture from the outset is vital, as late changes can be cumbersome and time-consuming. For instance, Meta has announced significant architectural updates for React Native that have yet to be implemented in a stable release.
Make technology choices based on your specific needs rather than current trends. While languages like Go and Rust are popular, C/C++ remains a strong option for producing lightweight binaries.
Section 2.5: Automate Repetitive Tasks
The DRY (Don't Repeat Yourself) principle encourages programmers to write maintainable code by reusing code segments and automation scripts. If you're performing a repetitive task manually, others on your team likely are too. Invest time in creating scripts to automate these processes.
Automation is a stepping stone toward implementing DevOps principles in your projects, making it essential to consider before engaging in repetitive manual tasks.
Conclusion
Best practices in programming are not rigid rules but rather a collection of widely recognized techniques that enhance the quality of software development. The practices discussed in this guide can be adapted based on individual experiences. Combine these insights with your own to cultivate a personalized set of best practices for daily coding tasks.
The second video title is "Write BETTER Code! 7 Tips to Improve Your Programming Skills" — This video offers practical tips for refining your coding abilities.