Unleashing Python's Potential: 3 Key Tricks for Developers
Written on
Mastering the 3 Essential Tricks for Coding Success 🚀💻
In the realm of Python programming, there are three fundamental tricks that every developer should be familiar with to enhance their coding skills.
Private Keyword
In Python, prefixing variable or method names with double underscores signifies that these members are meant to be private. This practice is referred to as "name mangling." Access to these private members is limited to the class in which they are defined. For instance:
class Gods:
def __init__(self, name) -> None:
self.__name = name
Attempting to access Gods("Zeus").__name will yield:
AttributeError: 'Gods' object has no attribute '__name'
When a member is marked private with the __ prefix, Python internally renames it, incorporating the class name as a prefix to prevent accidental name clashes with subclasses.
However, it's still possible to access and modify private members, though this practice is generally discouraged as it contravenes encapsulation principles:
gods = Gods("Zeus")
print(gods._Gods__name) # Outputs: Zeus
gods._Gods__name = "Poseidon"
print(gods._Gods__name) # Outputs: Poseidon
While this method grants access, it can lead to unexpected behavior. It's advisable to use public methods (getters and setters) for a more maintainable approach.
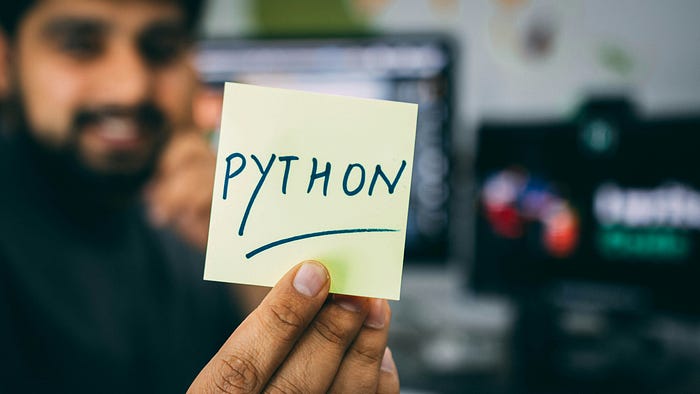
Dictionary Iteration Using Underscore
Python dictionaries are powerful structures for storing and retrieving data through key-value pairs. When iterating over these pairs, utilizing the underscore _ as a placeholder for the key can enhance code clarity and efficiency:
just_dict = dict(James="Yellow", Anna="Black", Dora="Brown", Sara="Green", John="Blue")
for _, color in just_dict.items():
print(color)
Output:
Yellow
Black
Brown
Green
Blue
In this example, the underscore indicates that the key is not utilized within the loop, thus making the code more concise and emphasizing that only the values are relevant during iteration.
Python Ternary Operators
Ternary operators in Python provide a streamlined way to express conditional statements succinctly:
result_if_true if condition else result_if_false
This expression evaluates a condition; if true, it returns result_if_true; otherwise, it returns result_if_false.
# Example 1
is_sunny = True
weather = "Good" if is_sunny else "Bad"
print(weather) # Outputs: Good
# Example 2
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num**2 if num % 2 == 0 else num for num in numbers]
print(squared_numbers) # Outputs: [1, 4, 3, 16, 5]
# Example 3
def get_discount(price, is_member):
return price * 0.8 if is_member else price
regular_price = 100
member_price = get_discount(regular_price, True)
non_member_price = get_discount(regular_price, False)
print(f"Member Price: {member_price}, Non-Member Price: {non_member_price}")
While ternary operators can be beneficial, it's important not to overuse them. They are best suited for simple conditions; complex scenarios might lead to confusion. Use them like a seasoning in your code—just a pinch can enhance flavor, but too much can overwhelm.
Chapter 2 Title: Exploring Practical Python Techniques
The first video titled "Unleashing the Power of Python: Solving 'Maximum Strength of a Group' Problem" dives deep into solving a specific problem using Python. It showcases practical applications of the language's capabilities.
The second video, "Unleash the Power of Grok AI: Your Complete Beginner's Guide (X.AI)," provides a comprehensive introduction to Grok AI, making it an excellent resource for newcomers looking to understand this powerful tool.
What do you think was the most valuable tip presented here? Would you like to explore more about private and public access modifiers? Do you frequently use ternary operations in your coding practices? Feel free to share your experiences and insights in the comments below.