Mastering Animation Events with Framer Motion in React
Written on
Understanding Framer Motion Animation Events
The Framer Motion library simplifies the process of implementing animations in React applications. In this article, we will explore how to effectively utilize animation events within Framer Motion.
Listening to Animation Events
Framer Motion allows us to respond to various animation events emitted from motion components. For instance, you can set up an onUpdate function to monitor the ongoing animation. Below is an example:
import React from "react";
import { motion } from "framer-motion";
function onUpdate(latest) {
console.log(latest.x, latest.opacity);
}
export default function App() {
return (
<motion.div
onUpdate={onUpdate}
animate={{ x: 100, opacity: 0 }}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
In this code, the onUpdate function logs the x-coordinate and opacity values during the animation.
Animation Start Event
To execute a function when an animation begins, we can use the onAnimationStart prop. Here’s how you might implement it:
import React from "react";
import { motion } from "framer-motion";
function onStart() {
console.log("Animation started");
}
export default function App() {
return (
<motion.div
onAnimationStart={onStart}
animate={{ x: 100, opacity: 0 }}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
This code snippet allows us to log a message when the animation begins.
Animation Completion Event
Similarly, the onAnimationComplete prop can be utilized to run a function once the animation is finished:
import React from "react";
import { motion } from "framer-motion";
function onComplete() {
console.log("Animation completed");
}
export default function App() {
return (
<motion.div
onAnimationComplete={onComplete}
animate={{ x: 100, opacity: 0 }}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
This setup allows you to log a message upon animation completion.
Hovering and Tapping Events
Framer Motion also supports hover events. We can listen for when the hover begins using the onHoverStart prop:
import React from "react";
import { motion } from "framer-motion";
export default function App() {
return (
<motion.div
onHoverStart={() => console.log("Hover starts")}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
When hovering over the component, "Hover starts" will be logged to the console.
Hover End Event
To detect when the hover ends, we can use the onHoverEnd prop:
import React from "react";
import { motion } from "framer-motion";
export default function App() {
return (
<motion.div
onHoverEnd={() => console.log("Hover ends")}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
This will log a message when the mouse leaves the component.
Tap Events
For capturing tap events, the onTap prop is available:
import React from "react";
import { motion } from "framer-motion";
function onTap(event, info) {
console.log(info.point.x, info.point.y);
}
export default function App() {
return (
<motion.div
onTap={onTap}
style={{ backgroundColor: "red", width: 100, height: 100 }}
></motion.div>
);
}
Here, tapping the component will log the coordinates of the tap.
Conclusion
Framer Motion provides a robust way to listen for various animation events, enhancing interactivity within your React applications.
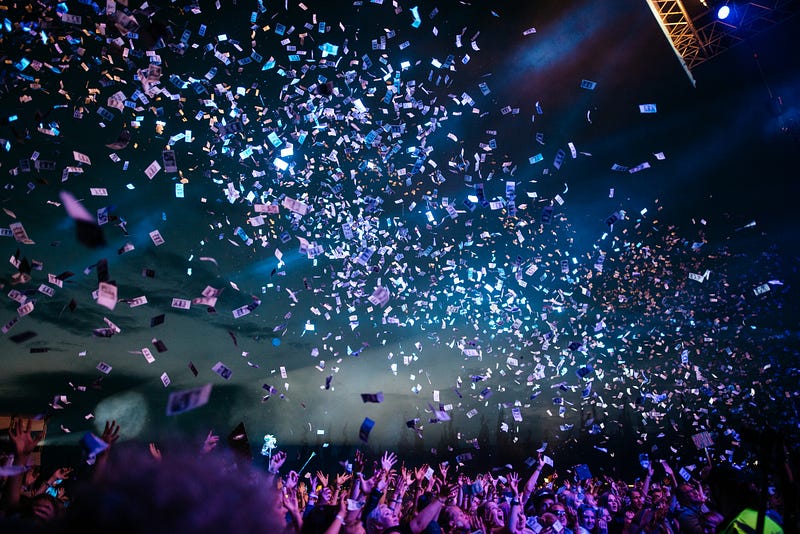
Chapter 2: Video Tutorials on Framer Motion
Explore further with these informative videos that delve into advanced animation techniques using Framer Motion.
This video covers complex animations using Framer Motion and the useAnimate hook, demonstrating how to achieve stunning effects in your React applications.
In this tutorial, you will learn about tap and animation events in Framer Playground, providing a practical approach to mastering these features.